Blazor offers two primary modes of rendering: Client-side and Server-side.
Client-side rendering, also known as Blazor WebAssembly, involves running the application code directly in the user’s browser. From a newbie perspective WASM executes as Client-side rendering, which means it works like other popular frameworks like React or Angular.
Yeah, but not exactly.
“WebAssembly is a low-level assembly-like language with a compact binary format that runs with near-native performance and provides languages such as C/C++, C# and Rust with a compilation target so that they can run on the web”. ~ Mozilla Docs
So this approach provides a highly responsive user experience as the application executes on the client side without frequent server round trips. However, it may require a larger initial load, as the WASM package is about 8 MB and can be less SEO-friendly than server-side rendering.

.NET 8 WASM package size
Source: Dawid ”Devinczi” Slysz
On the other hand, Interactive Server-side Rendering, known as Blazor Server, processes the application logic on the web server. It sends UI updates to the client over a SignalR (WebSocket) connection, minimizing the amount of code sent to the browser. This mode often results in faster load times since only the necessary UI updates are transmitted, but it can suffer from latency issues during user interactions due to the back-and-forth communication between the client and server.
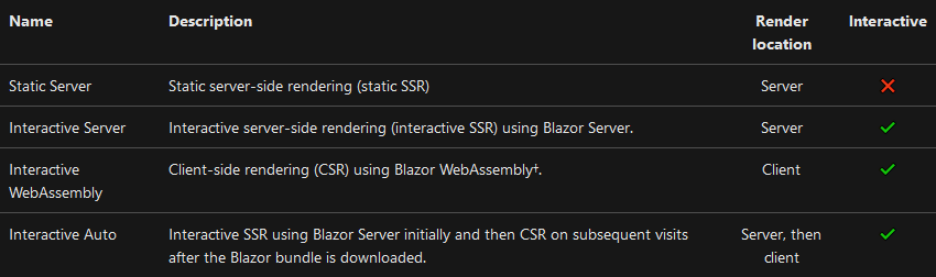
NET Attributes overview
Source: https://learn.microsoft.com/en-us/aspnet/core/blazor/components/render-modes?view=aspnetcore-8.0
Each render mode presents unique pros and cons:
Default – Blazor Static Server-side Render Mode:
Pros: SEO-friendly, Returns pure HTML in response
Cons: At base no interop with JavaScript (can be bypassed)
Blazor StreamRendering
@attribute [StreamRendering(true)]
Pros: Allows page to execute long running tasks (firstly returns HTML with placeholders and after that fill website with data), SEO-friendly (e.g. Youtube uses stream rendering)
Cons: Do not have 🙂
Blazor Interactive Server Render Mode:
@rendermode @(new InteractiveServerRenderMode(prerender: false));
Pros: Faster initial load, server-side processing, Availability of JavaScript Interop in method OnAfterRender()
Cons: Latency in user interactions, Scalability challenges with many users
Blazor WebAssembly:
@rendermode @(new InteractiveWebAssemblyRenderMode(prerender: false));
Pros: Client-side processing, No server dependency post load
Cons: Slower initial load due to downloading the app and the .NET runtime
Auto Render Mode:
@rendermode @(new InteractiveAutoRenderMode(prerender: false));
Pros: Combines faster initial load with client-side interactions
Cons: Complexity in setup, potential fallback to server rendering if client resources are insufficient
InteractiveAutoRenderMode To Rescue “All” Problems
Auto Render Mode in Blazor combines the benefits of Blazor Server and Blazor WebAssembly, offering a swift initial load followed by seamless client-side rendering. It starts with server-side rendering for rapid page load, then transitions to client-side rendering for interactive operations. This mode optimizes performance, grants particular control via @rendermode directive, and offers simplified setup through a structured approach. It’s a smart blend that enhances user experience and developer efficiency in .NET 8.
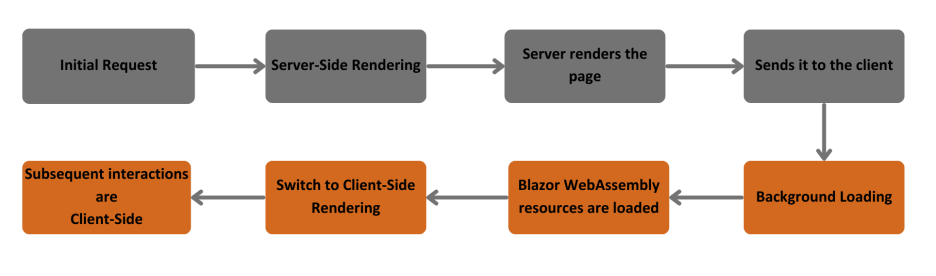
Flow of InteractiveAutoRenderMode
Source: Dawid ”Devinczi” Slysz
Potential Downsides
Auto Render Mode in Blazor is indeed a game-changer as it seeks to blend the advantages of both server-side and client-side rendering, offering a balanced rendering experience. However, like any technology, it comes with its set of limitations that could potentially impact its implementation in certain scenarios.
Auto Render Mode in Blazor has some considerations to keep in mind:
Complexity in Configuration: Setting up Auto Render Mode may involve specific configurations, adding complexity, especially for developers new to Blazor. It requires separate projects for components running via WebAssembly to prevent unnecessary server downloads to the browser.
Fixed Render Mode Post Decision: Once the render mode decision is made (either server-side or client-side), it remains fixed for the component’s lifetime. There’s no dynamic switching based on changing conditions during the user session.
Performance Impact: While Auto Render Mode aims for fast decision-making, there may be a slight delay as it checks for WebAssembly resources. Initial implementations used timeout checks, later updated to consider cached WebAssembly resources, with fallbacks if checks fail.
Dependency on Client Resources and Network Speed: The transition to client-side rendering depends heavily on client resources and network speed. Slow client machines or sluggish networks can delay the transition, impacting app performance.
Conclusion
Overall, during development, choosing the type of rendering between Static-SSR through Interactive-SSR and CSR seems powerful.
In my honest opinion, despite all challenges mentioned before, Auto Render Mode in Blazor represents a significant advancement in rendering technology, enhancing user experience and developer efficiency in .NET 8.